Grouping
RadListView provides you with the functionality to programmatically group its data.
This can be achieved through adding group descriptors to the RadListView.GroupDescriptors collection. The following list shows the available types of group descriptors:
-
PropertyGroupDescriptor : Enables you to group the data by a property value from the data model of the items. This descriptor exposes the following properties:
- PropertyName : Gets or sets the string name of the property you want to group by.
- SortOrder : Changes the sort order in each group to Ascending or Descending.
DelegateGroupDescriptor : Enables you to group by a custom key(e.g. some complex expression combining two or more properties) instead of being limited by the value of a single property of your item. This descriptor exposes the following properties:
- KeyLookup : Gets or sets the IKeyLookup instance used to retrieve the group key for each data item.
- SortOrder : Changes the sort order in each group to Ascending or Descending.
Group Header Scroll Behavior
By default the topmost group header is pinned to the top of the viewport until another group header reaches its position. This happens when you scroll up/down. In this case, the next group header pins to the top and the process repeats until you get the end or the begining of the scrollbar. This behavior can be disabled by setting the GroupHeaderDisplayMode property of RadListView. The following modes are available:
Frozen: The first group header stays static until the next group header takes its place during scrolling. This is the default value.
Scrollable: The group header is scrolling along with the other items in the view.
Example
The following example demonstrates how you can use DelegateGroupDescriptor.
Example 1: Defining the item model and group key lookup
public class Item
{
public string Name { get; set; }
public double Id { get; set; }
}
public class Key : IKeyLookup
{
public object GetKey(object instance)
{
return (instance as Item).Id % 2;
}
}
Example 2: Populating with data
public MainWindow()
{
this.InitializeComponent();
List<Item> list = new List<Item>();
for (int i = 0; i < 10; i++)
{
list.Add(new Item { Id = i, Name = "Mr." + i });
}
this.DataContext = list;
}
Example 3: Adding a group descriptor in XAML
<telerikData:RadListView ItemsSource="{Binding}">
<telerikData:RadListView.Resources>
<local:Key x:Key="key" />
</telerikData:RadListView.Resources>
<telerikData:RadListView.ItemTemplate>
<DataTemplate>
<TextBlock Text="{Binding Name}" />
</DataTemplate>
</telerikData:RadListView.ItemTemplate>
<telerikData:RadListView.GroupDescriptors>
<dataCore:DelegateGroupDescriptor SortOrder="Descending" KeyLookup="{StaticResource key}" />
</telerikData:RadListView.GroupDescriptors>
</telerikData:RadListView>
Example 4: Telerik namespace definitions
xmlns:telerikData="using:Telerik.UI.Xaml.Controls.Data"
xmlns:dataCore="using:Telerik.Data.Core"
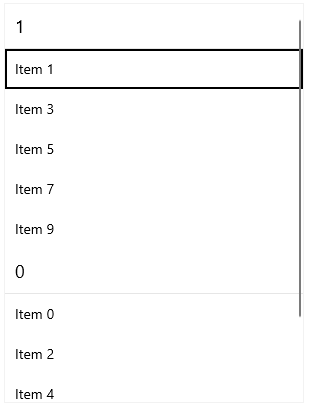