Incremental Loading
The RadListView incremental loading feature allows you to load the data in separate batches when you reach the end of the scrollbar or click on a button.
To enable incremental loading, the ItemsSource collection of RadListView should implement the ISupportIncrementalLoading interface. You can also take advantage of the IncrementalLoadingCollection which is our default implementation.
The IncrementalLoadingMode property specifies how new items are requested. Two loading modes are supported:
- Automatic: Scrolling to the end of the control automatically loads more items.
-
Explicit: The user has to explicitly request more items by click/tap on the button.
The RadListView also supports LoadMoreDataCommand that by default is executed to load new data. Read more about commands in the Commands article.
Using IncrementalLoading Collection
The following example demonstrates how to enable data virtualization using the incremental loading functionality. This approach works for both Automatic and Explicit loading modes.
Example 1: Creating data models
Example 2: Defining RadListView in XAML
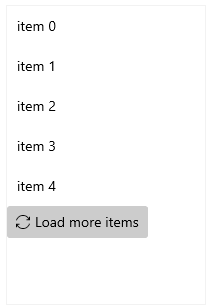
Using LoadMoreDataCommand
This example demonstrates how to utilize LoadMoreDataCommand to load items. The approach works for both Automatic and Explicit loading modes.