Nested Properties
PropertyGrid can show nested property fields that can be expanded and edited.
To enable the nested property definitions, set the NestedPropertiesVisibility
property of RadPropertyGrid
to Visible
. That way you will be able to modify any editable child properties of reference types.
Creating the model
public class Order
{
public DateTime OrderDate { get; set; }
public DateTime ShippedDate { get; set; }
public string ShipAddress { get; set; }
public string ShipName { get; set; }
public string ShipCountry { get; set; }
public string ShipPostalCode { get; set; }
public Employee Employee { get; set; }
}
public class Employee
{
public string FirstName { get; set; }
public string LastName { get; set; }
public string Title { get; set; }
public string HomePhone { get; set; }
public Department Department { get; set; }
}
public class Department
{
public int Id { get; set; }
public string Country { get; set; }
}
Setting up the data
this.propertyGrid.Item = new Order()
{
OrderDate = new DateTime(1996, 7, 5),
ShippedDate = new DateTime(1996, 8, 16),
ShipAddress = "Luisenstr. 48",
ShipCountry = "Germany",
ShipName = "Toms Spezialitaten",
ShipPostalCode = "44087",
Employee = new Employee()
{
FirstName = "Nancy",
LastName = "Davolio",
Title = "Sales Representative",
HomePhone = "(206) 555-9857",
Department = new Department()
{
Country = "USA",
Id = 1
}
},
};
Defining RadPropertyGrid
<telerikControls:RadPropertyGrid x:Name="propertyGrid" NestedPropertiesVisibility="Visible"/>
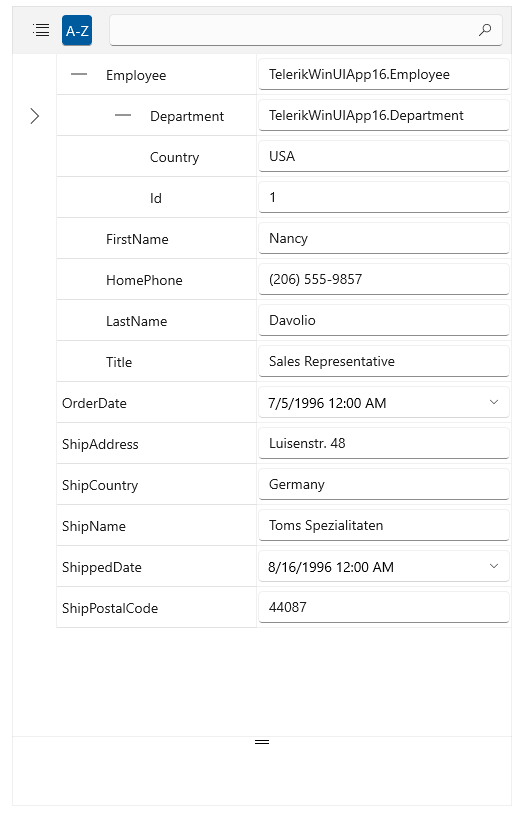
Defining Nested Property Definitions Manually
To define nested properties for a PropertyDefinition object, use its NestedProperties
property.
Setting up nested properties manually
<telerikControls:RadPropertyGrid>
<telerikControls:RadPropertyGrid.PropertyDefinitions>
<propertyGrid:PropertyDefinition Binding="{Binding Employee}">
<propertyGrid:PropertyDefinition.NestedProperties>
<propertyGrid:PropertyDefinition DisplayName="First name" Binding="{Binding FirstName}"/>
</propertyGrid:PropertyDefinition.NestedProperties>
</propertyGrid:PropertyDefinition>
</telerikControls:RadPropertyGrid.PropertyDefinitions>
</telerikControls:RadPropertyGrid>