Data Validation
The PropertyGrid control supports data validation that allows you to tell if the input is within the expected values or not.
The validation is supported only when the EditMode property is set to Single
.
To validate the edited value, use the Validating
event. This will allow you to set the IsValid
property of the event arguments to false
when needed. The label of the invalid field will be highlighted with a red foreground and an exclamation mark icon will be displayed next to the editor. The exclamation mark icon has a tooltip displayed on mouse over.
To set a display message that will be shown in the tooltip, use the ValidationResults
property of the Validated
event of RadPropertyGrid
.
The following example shows how to setup the PropertyGrid control and implement data validation using the events.
Creating the item model
public class Employee
{
public string FirstName { get; set; }
public string LastName { get; set; }
public double Salary { get; set; }
public bool IsManager { get; set; }
public DateTime HireDate { get; set; }
}
Setting up the item
this.propertyGrid.Item = new Employee()
{
FirstName = "John",
LastName = "Doe",
Salary = 10000,
HireDate = DateTime.Today
};
Subscribing to the validation events
<telerikControls:RadPropertyGrid x:Name="propertyGrid"
EditMode="Single"
Validating="RadPropertyGrid_Validating"
Validated="RadPropertyGrid_Validated">
Implementing data validation
private void RadPropertyGrid_Validating(object sender, Telerik.UI.Xaml.Controls.Data.PropertyGrid.PropertyGridValidatingEventArgs e)
{
var propertyDefinition = (PropertyDefinition)e.Field.DataContext;
if (propertyDefinition.DisplayName == "FirstName" && string.IsNullOrEmpty(propertyDefinition.Value.ToString()))
{
propertyNameToErrorMessageDict[propertyDefinition.DisplayName] = "First name cannot be empty.";
e.IsValid = false;
}
else if (propertyDefinition.DisplayName == "Salary" && (double)propertyDefinition.Value < 1000)
{
propertyNameToErrorMessageDict[propertyDefinition.DisplayName] = "Salary cannot be less than 1000.";
e.IsValid = false;
}
}
private void RadPropertyGrid_Validated(object sender, Telerik.UI.Xaml.Controls.Data.PropertyGrid.PropertyGridValidatedEventArgs e)
{
var propertyDefinition = (PropertyDefinition)e.Field.DataContext;
if (propertyNameToErrorMessageDict.ContainsKey(propertyDefinition.DisplayName))
{
string errorMessage = propertyNameToErrorMessageDict[propertyDefinition.DisplayName];
e.ValidationResults.Add(new ValidationResult(errorMessage));
propertyNameToErrorMessageDict.Remove(propertyDefinition.DisplayName);
}
}
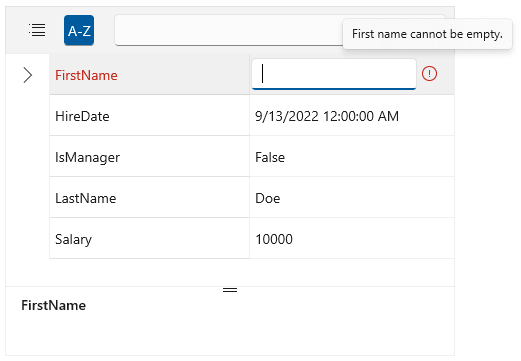