Customizing Items Appearance
The content of items in CollectionEditor control can be customized by overriding the ToString
method of the underlying data item's class or by using the ItemTemplate
property of RadCollectionEditor
.
Using ItemTemplate
The ItemTemplate
property allows you to define a DataTemplate
in order to replace the default appearance of the collection item visuals.
Setting the ItemTemplate
<telerikControls:RadCollectionEditor>
<telerikControls:RadCollectionEditor.ItemTemplate>
<DataTemplate>
<StackPanel Orientation="Horizontal">
<Rectangle Width="16" Height="16" Fill="#FFD661"/>
<TextBlock Text="{Binding FirstName}" Margin="5 0 0 0" />
<TextBlock Text="{Binding LastName}" Margin="3 0 0 0"/>
</StackPanel>
</DataTemplate>
</telerikControls:RadCollectionEditor.ItemTemplate>
</telerikControls:RadCollectionEditor>
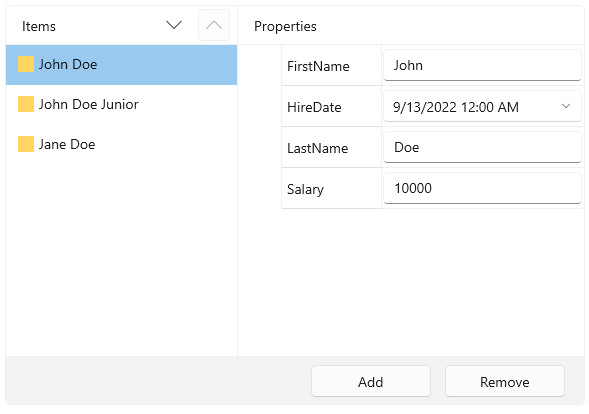
Overriding ToString
Overriding the ToString
method of the underlying data item's class allows you to easily replace the default content of the items.
The following example shows how to override ToString
and populate the RadCollectionEditor
with items.
Creating the data model
public class Employee
{
public string FirstName { get; set; }
public string LastName { get; set; }
public double Salary { get; set; }
public DateTime HireDate { get; set; }
public override string ToString()
{
return this.FirstName + " " + this.LastName;
}
}
Setting up the data
this.collectionEditorPicker.Source = new BindableCollection<Employee>()
{
new Employee() { FirstName = "John", LastName = "Doe", Salary = 10000, HireDate = DateTime.Today },
new Employee() { FirstName = "John", LastName = "Doe Junior", Salary = 10000, HireDate = DateTime.Today },
new Employee() { FirstName = "Jane", LastName = "Doe", Salary = 10000, HireDate = DateTime.Today },
};
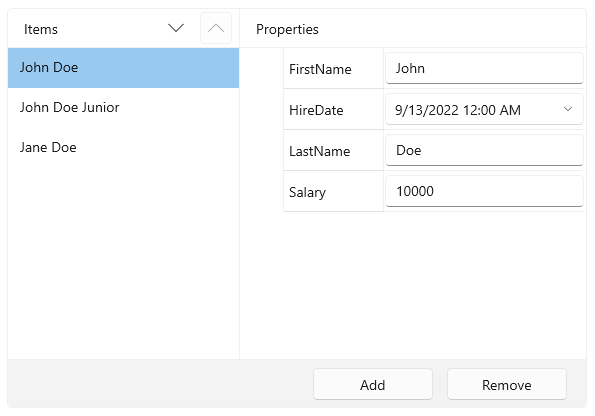