Grouping
RadAutoSuggestBox allows you to visualize groups when ICollectionView with GroupDescriptors is used as an ItemsSource.
The following example shows how to populate the ItemsSource of RadAutoSuggestBox with an ICollectionView and enabling the grouping feature of the control. The feature shows group headers and organizes the items in the drop down of the control by groups. To enable the headers, set the GroupStyle property of RadAutoSuggestBox.
Read more about the GroupStyle object expected by the GroupStyle property in MSDN.
Example 1: Define RadAutoSuggestBox and enable grouping
<telerik:RadAutoSuggestBox x:Name="radAutoSuggestBox"
DisplayMemberPath="Name"
TextMemberPath="Name"
TextChanged="RadAutoSuggestBox_TextChanged">
<telerik:RadAutoSuggestBox.GroupStyle>
<GroupStyle>
<GroupStyle.HeaderTemplate>
<DataTemplate>
<TextBlock Text="{Binding Name}"
FontSize="18"
Foreground="#27C106"
Margin="5" />
</DataTemplate>
</GroupStyle.HeaderTemplate>
</GroupStyle>
</telerik:RadAutoSuggestBox.GroupStyle>
</telerik:RadAutoSuggestBox>
Example 2: Create model for the items
public class SuggestionInfo
{
public string Name { get; set; }
public string GroupKey { get; set; }
}
Example 3: Populate the ItemsSource with ICollectionView object and prepare a filter
public MainWindow()
{
InitializeComponent();
var source = new ObservableCollection<SuggestionInfo>();
for (int i = 0; i < 3; i++)
{
string currentGroup = "G" + i;
for (int k = 0; k < 4; k++)
{
source.Add(new SuggestionInfo() { Name = "Item " + k, GroupKey = currentGroup });
}
}
var collectionView = CollectionViewSource.GetDefaultView(source);
collectionView.GroupDescriptions.Add(new PropertyGroupDescription("GroupKey"));
collectionView.Filter = new Predicate<object>(FilterItem);
this.radAutoSuggestBox.ItemsSource = collectionView;
}
public bool FilterItem(object value)
{
return ((SuggestionInfo)value).Name.ToLowerInvariant().Contains(this.radAutoSuggestBox.Text.ToLowerInvariant());
}
Example 4: Trigger the ICollectionView filtering on TextChanged
private void RadAutoSuggestBox_TextChanged(object sender, Telerik.Windows.Controls.AutoSuggestBox.TextChangedEventArgs e)
{
if (e.Reason == TextChangeReason.UserInput)
{
var collectionView = (ICollectionView)this.radAutoSuggestBox.ItemsSource;
collectionView.Refresh();
}
}
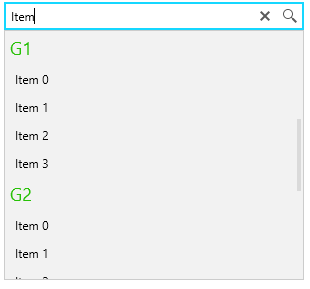