Data Binding to Collection
This article shows how to use RadChat in MVVM scenario by data binding its items source to a collection of view models.
To data bind the messages list you can use the DataSource and MessageConverter properties of the RadChat control.
The DataSource property expects a collection of business objects containing information about the messages.
The MessageConverter allows you to use an implementation of the IMessageConverter interface. This is used to convert between the business object models and the RadChat message models. The ConvertItem method should convert a business object to a message object and ConvertMessage should convert a message object to a business object.
The following example shows how to define custom models and use the converter.
Example 1: Defining the messages view model
public class TextMessageModel
{
public string Text { get; set; }
public Author Author { get; set; }
public DateTime CreationDate { get; set; }
}
Example 2: Defining the main view model and populating the data source with data
public class MainViewModel
{
public ObservableCollection<TextMessageModel> Messages { get; set; }
public Author CurrentAuthor { get; private set; }
public MainViewModel()
{
this.CurrentAuthor = new Author("Martin");
this.Messages = new ObservableCollection<TextMessageModel>();
var otherAuthor = new Author("Virtual Assistant");
this.Messages.Add(new TextMessageModel() { Text = "Hello Martin,", Author = otherAuthor, CreationDate = DateTime.Now });
this.Messages.Add(new TextMessageModel() { Text = "I am your Virtual Assistant", Author = otherAuthor, CreationDate = DateTime.Now });
this.Messages.Add(new TextMessageModel() { Text = "How can I help you?", Author = otherAuthor, CreationDate = DateTime.Now });
this.Messages.Add(new TextMessageModel() { Text = "Hi", Author = this.CurrentAuthor, CreationDate = DateTime.Now.AddMinutes(5) });
this.Messages.Add(new TextMessageModel() { Text = "Show me the weather for this week", Author = this.CurrentAuthor, CreationDate = DateTime.Now.AddMinutes(5) });
}
}
Example 3: Implementing message converter
public class MessageConverter : IMessageConverter
{
public MessageBase ConvertItem(object item)
{
var messageModel = (TextMessageModel)item;
return new TextMessage(messageModel.Author, messageModel.Text, messageModel.CreationDate);
}
public object ConvertMessage(MessageBase message)
{
var textMessage = (TextMessage)message;
return new TextMessageModel()
{
Author = textMessage.Author,
Text = textMessage.Text,
CreationDate = textMessage.CreationDate
};
}
}
Example 4: Setting up the RadChat control
<telerik:RadChat CurrentAuthor="{Binding CurrentAuthor}" DataSource="{Binding Messages}">
<telerik:RadChat.DataContext>
<local:MainViewModel />
</telerik:RadChat.DataContext>
<telerik:RadChat.MessageConverter>
<local:MessageConverter />
</telerik:RadChat.MessageConverter>
</telerik:RadChat>
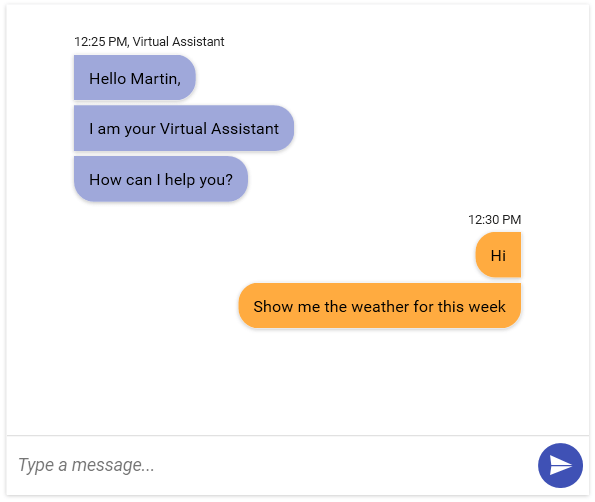
The example demonstrates how to work with text messages, but the same approach is also applicable for the other message types.