Data Binding
This article demonstrates how to bind the RadTabbedWindow control to a collection of business objects.
For the purposes of this example the control is bound to a collection of Tab objects. Please note that the class inherits from ViewModelBase which is the Telerik implementation of the INotifyPropertyChanged interface.
Example 1: The Tab class
Example 2: Create an ObservableCollection in the viewmodel
Example 3: Bind the ItemsSource property
As of R2 2019 SP1 the control's Items property (populated when setting the control's ItemsSource) is of type IList. Prior to this version of the controls, it was of type ObservableCollection
.
Please note that you need to replace the DataBinding namespace with your namespace.
Upon running the application, your RadTabbedWindow will now be populated with tabs as shown on Figure 1.
Figure 1: DataBound RadTabbedWindow
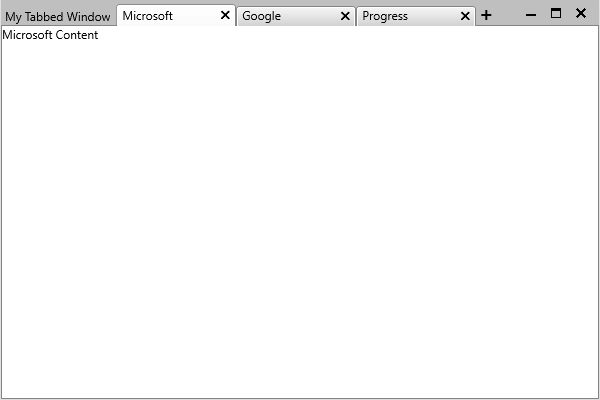